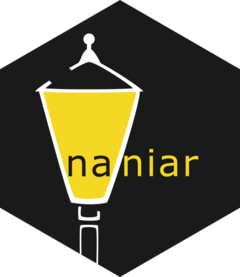
Replace values with NA based on some condition, for variables that meet some predicate
Source:R/scoped-replace-with-na.R
replace_with_na_if.Rd
Replace values with NA based on some condition, for variables that meet some predicate
Arguments
- data
Dataframe
- .predicate
A predicate function to be applied to the columns or a logical vector.
- condition
A condition required to be TRUE to set NA. Here, the condition is specified with a formula, following the syntax:
~.x {condition}
. For example, writing~.x < 20
would mean "where a variable value is less than 20, replace with NA".
Examples
dat_ms <- tibble::tribble(~x, ~y, ~z,
1, "A", -100,
3, "N/A", -99,
NA, NA, -98,
-99, "E", -101,
-98, "F", -1)
dat_ms
#> # A tibble: 5 × 3
#> x y z
#> <dbl> <chr> <dbl>
#> 1 1 A -100
#> 2 3 N/A -99
#> 3 NA NA -98
#> 4 -99 E -101
#> 5 -98 F -1
replace_with_na_if(data = dat_ms,
.predicate = is.character,
condition = ~.x == "N/A")
#> # A tibble: 5 × 3
#> x y z
#> <dbl> <chr> <dbl>
#> 1 1 A -100
#> 2 3 NA -99
#> 3 NA NA -98
#> 4 -99 E -101
#> 5 -98 F -1
replace_with_na_if(data = dat_ms,
.predicate = is.character,
condition = ~.x %in% common_na_strings)
#> # A tibble: 5 × 3
#> x y z
#> <dbl> <chr> <dbl>
#> 1 1 A -100
#> 2 3 NA -99
#> 3 NA NA -98
#> 4 -99 E -101
#> 5 -98 F -1
replace_with_na(dat_ms,
to_na = list(x = c(-99, -98),
y = c("N/A"),
z = c(-101)))
#> # A tibble: 5 × 3
#> x y z
#> <dbl> <chr> <dbl>
#> 1 1 A -100
#> 2 3 N/A -99
#> 3 NA NA -98
#> 4 -99 E -101
#> 5 -98 F -1