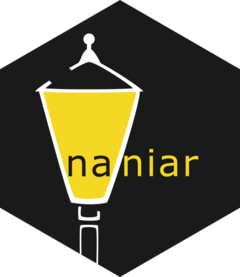
Replace all values with NA where a certain condition is met
Source:R/scoped-replace-with-na.R
replace_with_na_all.Rd
This function takes a dataframe and replaces all values that meet the condition specified as an NA value, following a special syntax.
Arguments
- data
A dataframe
- condition
A condition required to be TRUE to set NA. Here, the condition is specified with a formula, following the syntax:
~.x {condition}
. For example, writing~.x < 20
would mean "where a variable value is less than 20, replace with NA".
Examples
dat_ms <- tibble::tribble(~x, ~y, ~z,
1, "A", -100,
3, "N/A", -99,
NA, NA, -98,
-99, "E", -101,
-98, "F", -1)
dat_ms
#> # A tibble: 5 × 3
#> x y z
#> <dbl> <chr> <dbl>
#> 1 1 A -100
#> 2 3 N/A -99
#> 3 NA NA -98
#> 4 -99 E -101
#> 5 -98 F -1
#replace all instances of -99 with NA
replace_with_na_all(data = dat_ms,
condition = ~.x == -99)
#> # A tibble: 5 × 3
#> x y z
#> <dbl> <chr> <dbl>
#> 1 1 A -100
#> 2 3 N/A NA
#> 3 NA NA -98
#> 4 NA E -101
#> 5 -98 F -1
# replace all instances of -99 or -98, or "N/A" with NA
replace_with_na_all(dat_ms,
condition = ~.x %in% c(-99, -98, "N/A"))
#> # A tibble: 5 × 3
#> x y z
#> <dbl> <chr> <dbl>
#> 1 1 A -100
#> 2 3 NA NA
#> 3 NA NA NA
#> 4 NA E -101
#> 5 NA F -1
# replace all instances of common na strings
replace_with_na_all(dat_ms,
condition = ~.x %in% common_na_strings)
#> # A tibble: 5 × 3
#> x y z
#> <dbl> <chr> <dbl>
#> 1 1 A -100
#> 2 3 NA -99
#> 3 NA NA -98
#> 4 -99 E -101
#> 5 -98 F -1
# where works with functions
replace_with_na_all(airquality, ~ sqrt(.x) < 5)
#> # A tibble: 153 × 6
#> Ozone Solar.R Wind Temp Month Day
#> <int> <int> <dbl> <int> <int> <int>
#> 1 41 190 NA 67 NA NA
#> 2 36 118 NA 72 NA NA
#> 3 NA 149 NA 74 NA NA
#> 4 NA 313 NA 62 NA NA
#> 5 NA NA NA 56 NA NA
#> 6 28 NA NA 66 NA NA
#> 7 NA 299 NA 65 NA NA
#> 8 NA 99 NA 59 NA NA
#> 9 NA NA NA 61 NA NA
#> 10 NA 194 NA 69 NA NA
#> # ℹ 143 more rows